Revisiting last year’s challenge, let’s see how this year goes with GPT-4.
I used the same prompt from 2022:
Draw a Christmas tree using SwiftUI
Interestingly this time it included the Triangle
type and it was almost line-for-line the exact one I substituted in last year.
import SwiftUI
struct ContentView: View {
var body: some View {
ZStack {
// Background
Color.white.edgesIgnoringSafeArea(.all)
// Christmas Tree
VStack {
ForEach((1...5).reversed(), id: \.self) { i in
TreeLayer(width: CGFloat(i) * 60)
}
Rectangle()
.frame(width: 40, height: 50)
.foregroundColor(.brown)
}
}
}
}
struct TreeLayer: View {
var width: CGFloat
var body: some View {
ZStack {
Triangle()
.fill(Color.green)
.frame(width: width, height: width * 0.75)
// Add decorations here
}
}
}
struct Triangle: Shape {
func path(in rect: CGRect) -> Path {
var path = Path()
path.move(to: CGPoint(x: rect.midX, y: rect.minY))
path.addLine(to: CGPoint(x: rect.minX, y: rect.maxY))
path.addLine(to: CGPoint(x: rect.maxX, y: rect.maxY))
path.closeSubpath()
return path
}
}
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
And here’s the output for 2022 and 2023! 🎄😀
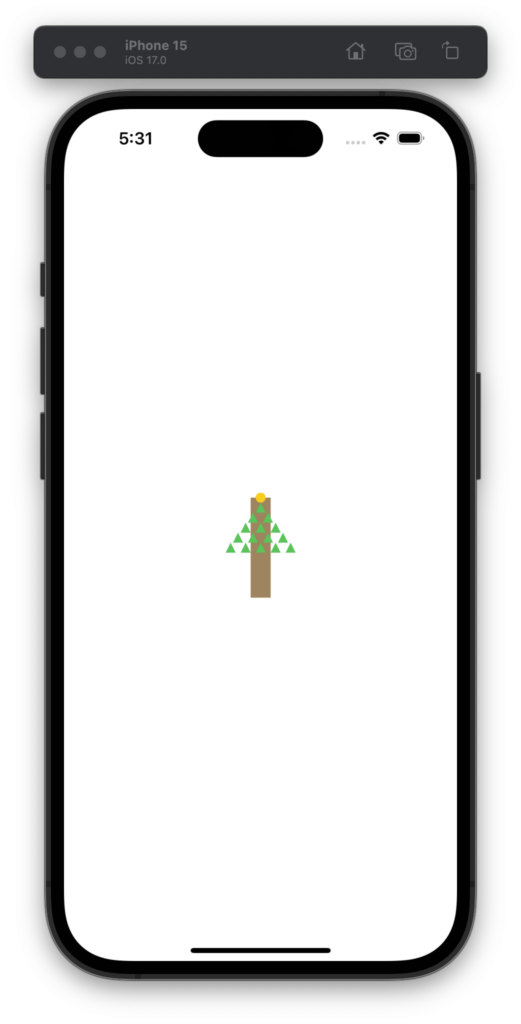
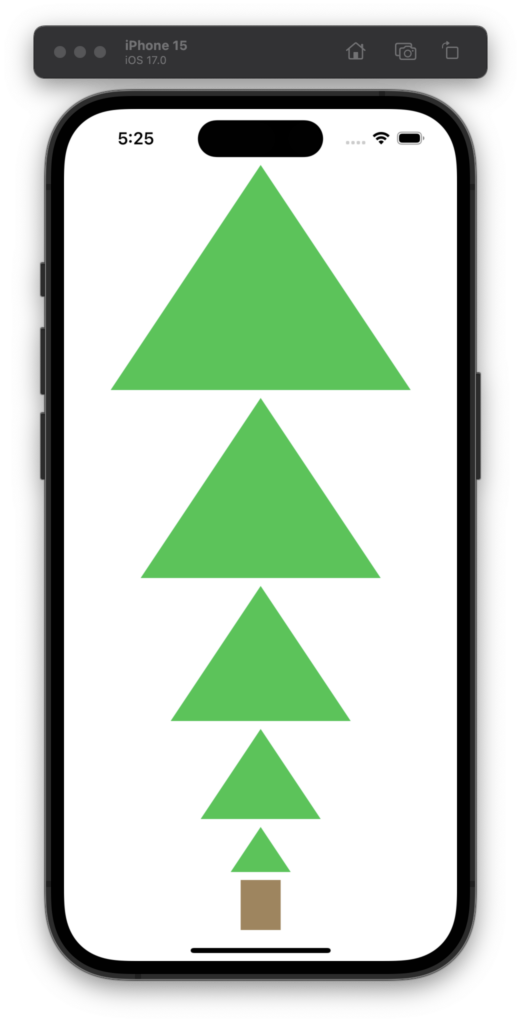