Over the past couple of years (2022, 2023) I’ve compared the output of the following prompt to ChatGPT:
Draw a Christmas tree using SwiftUI
For 2024 I chose the GPT-4o model, and this is what it output:
import SwiftUI
struct ChristmasTreeView: View {
var body: some View {
ZStack {
// Background color
Color.blue
.edgesIgnoringSafeArea(.all)
// Tree
VStack(spacing: -20) {
Triangle()
.fill(Color.green)
.frame(width: 150, height: 100)
Triangle()
.fill(Color.green)
.frame(width: 180, height: 120)
Triangle()
.fill(Color.green)
.frame(width: 210, height: 140)
// Tree trunk
Rectangle()
.fill(Color.brown)
.frame(width: 40, height: 60)
}
.offset(y: 50)
// Star on top
StarShape()
.fill(Color.yellow)
.frame(width: 40, height: 40)
.offset(y: -170)
// Decorations (Ornaments)
Circle()
.fill(Color.red)
.frame(width: 15, height: 15)
.offset(x: -40, y: -60)
Circle()
.fill(Color.yellow)
.frame(width: 15, height: 15)
.offset(x: 50, y: -30)
Circle()
.fill(Color.white)
.frame(width: 15, height: 15)
.offset(x: -30, y: 20)
Circle()
.fill(Color.blue)
.frame(width: 15, height: 15)
.offset(x: 40, y: 60)
}
}
}
// Triangle shape for the tree
struct Triangle: Shape {
func path(in rect: CGRect) -> Path {
Path { path in
path.move(to: CGPoint(x: rect.midX, y: rect.minY))
path.addLine(to: CGPoint(x: rect.minX, y: rect.maxY))
path.addLine(to: CGPoint(x: rect.maxX, y: rect.maxY))
path.closeSubpath()
}
}
}
// Star shape for the top of the tree
struct StarShape: Shape {
func path(in rect: CGRect) -> Path {
let points = [
CGPoint(x: 0.5, y: 0),
CGPoint(x: 0.61, y: 0.35),
CGPoint(x: 1, y: 0.35),
CGPoint(x: 0.68, y: 0.57),
CGPoint(x: 0.79, y: 0.91),
CGPoint(x: 0.5, y: 0.7),
CGPoint(x: 0.21, y: 0.91),
CGPoint(x: 0.32, y: 0.57),
CGPoint(x: 0, y: 0.35),
CGPoint(x: 0.39, y: 0.35)
].map { CGPoint(x: $0.x * rect.width, y: $0.y * rect.height) }
return Path { path in
path.move(to: points[0])
for point in points.dropFirst() {
path.addLine(to: point)
}
path.closeSubpath()
}
}
}
struct ChristmasTreeView_Previews: PreviewProvider {
static var previews: some View {
ChristmasTreeView()
}
}
Which generated:
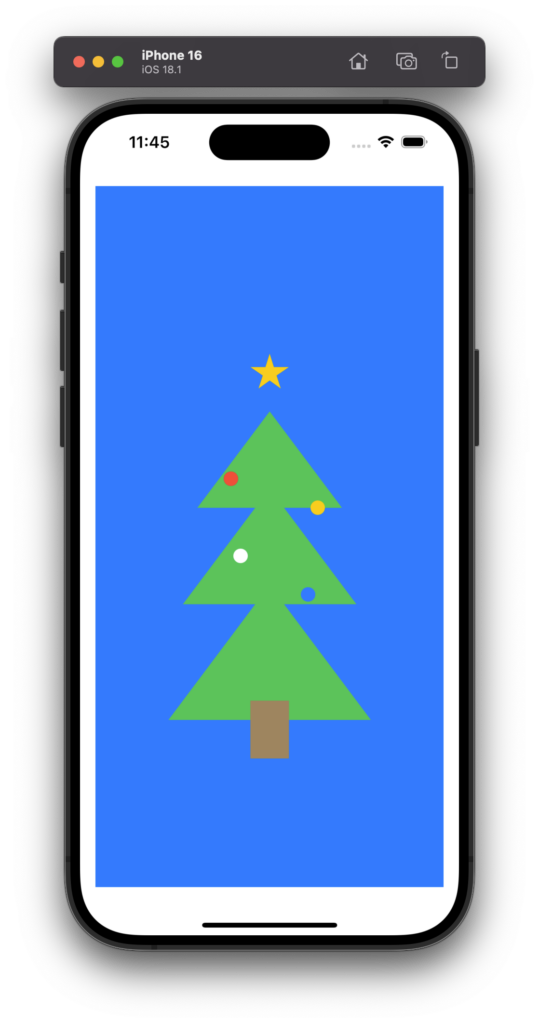
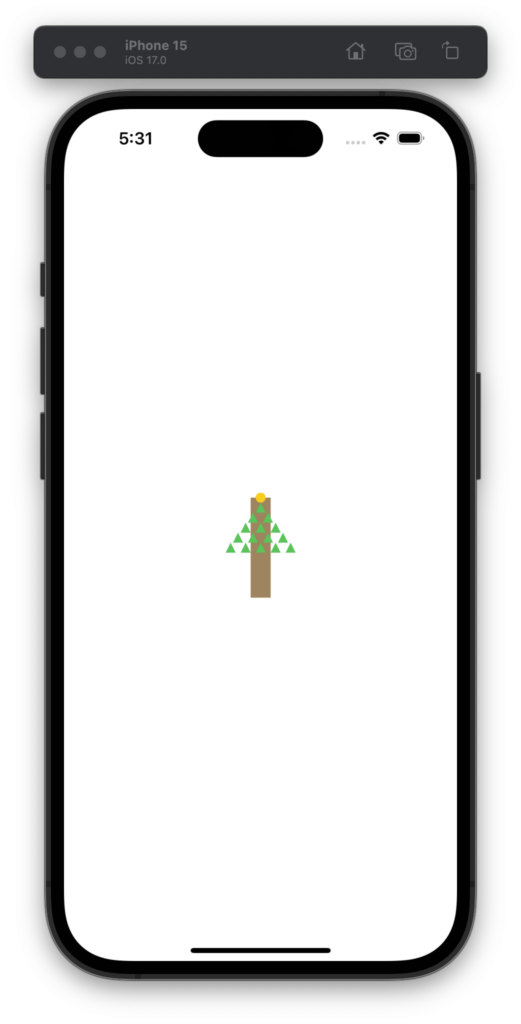
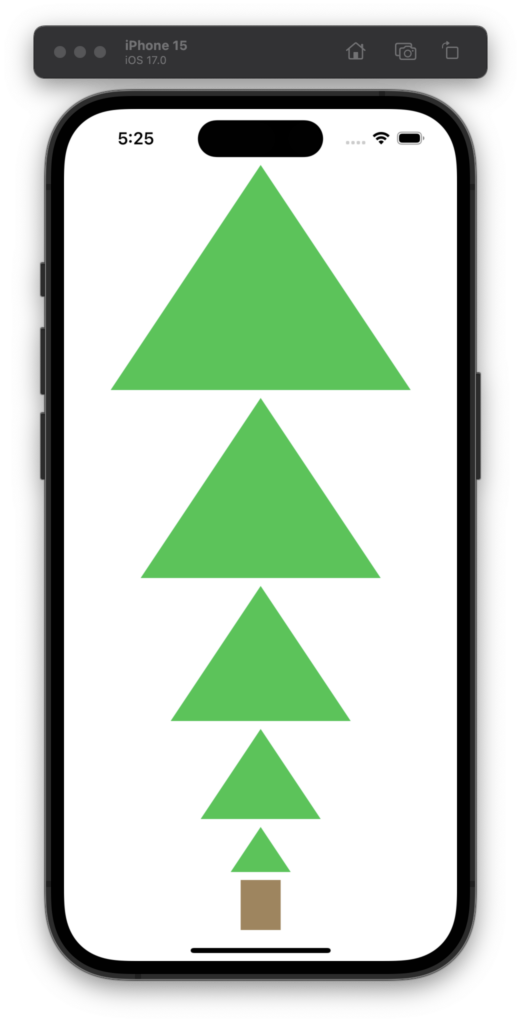
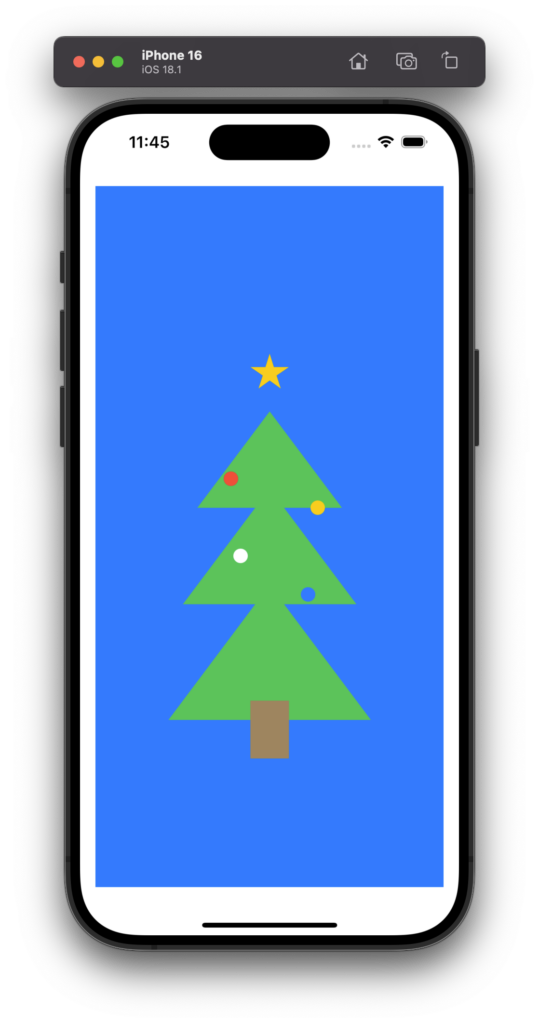
This is the best one yet! I didn’t have to add or change any code to make it work, it added ornaments and even a (floating 😀) star! 🎄